Smbus Library
SMBus Library
The SMBus I/O interface is a two-wire, bi-directional serial bus. The System Management Bus is compatible with the I2C serial bus.
SMBus module is available with a number of Silicon Laboratories 8051 MCU models. mikroC PRO for 8051 provides library which supports the master SMBus mode.
Library Routines
SMBus1_Init
Prototype | void SMBus1_Init(unsigned long clock); |
---|---|
Returns | Nothing. |
Description | Initializes SMBus with desired |
Requires | Library requires SMBus module. |
Example |
SMBus1_Start
Prototype | char SMBus1_Start(); |
---|---|
Returns | If there is no error function returns 0, otherwise returns 1. |
Description | Determines if SMBus bus is free and issues START signal. |
Requires | SMBus must be configured before using this function. See SMBus1_Init. |
Example |
SMBus1_Repeated_Start
Prototype | void SMBus1_Repeated_Start(void); |
---|---|
Returns | Nothing. |
Description | Issues repeated START signal. |
Requires | SMBus must be configured before using this function. See SMBus1_Init. |
Example |
The Power Management Bus (PMBus) is an open standard power-management protocol with fully defined commands that support communication with power converters and other digital power-management devices and host processors in a power system. The protocol is implemented over the industry-standard SMBus serial interface and enables programming. Jan 17, 2021 Starting with v0.2, the smbus2 library also has support for combined read and write transactions. I2crdwr is not really a SMBus feature but comes in handy when the master needs to: read or write bulks of data larger than SMBus' 32 bytes limit. Write some data and then read from the slave with a repeated start and no stop bit between. C and SMBus Subsystem. I 2 C (or without fancy typography, “I2C”) is an acronym for the “Inter-IC” bus, a simple bus protocol which is widely used where low data rate communications suffice. Since it’s also a licensed trademark, some vendors use another name (such as “Two-Wire Interface”, TWI) for the same bus. VENDOR/CONTRACTOR INFORMATION. This guide is intended to assist vendors and contractors interested in doing business with the California State University, Dominguez Hills through the Office of Procurement and Contracts.
SMBus1_Busy
Prototype | char SMBus1_Busy(); |
---|---|
Returns | Returns 0 if SMBus start sequence is finished, 1 if SMBus start sequence is not finished. |
Description | Signalizes the status of SMBus bus. |
Requires | SMBus must be configured before using this function. See SMBus1_Init. |
Example |
SMBus1_Read
Prototype | char SMBus1_Read(char nack); |
---|---|
Returns | Returns one byte from the slave. |
Description | Reads one byte from the slave, and sends acknowledge signal if parameter |
Requires | SMBus must be configured before using this function. See SMBus1_Init. Also, START signal needs to be issued in order to use this function. See SMBus1_Start. |
Example | Read data and send not acknowledge signal: |
SMBus1_Write
Prototype | void SMBus1_Write(char data_); |
---|---|
Returns | Nothing. |
Description | Sends data byte (parameter |
Requires | SMBus must be configured before using this function. See SMBus1_Init. Also, START signal needs to be issued in order to use this function. See SMBus1_Start. |
Example |
SMBus1_Status
Prototype | char SMBus1_Status(); |
---|---|
Returns | Returns value of status register. |
Description | Returns status of SMBus. |
Requires | SMBus must be configured before using this function. See SMBus1_Init. |
Example |
SMBus1_Stop
Prototype | void SMBus1_Stop(); |
---|---|
Returns | Nothing. |
Description | Issues STOP signal to SMBus operation. |
Requires | SMBus must be configured before using this function. See SMBus1_Init. |
Example |
SMBus1_Close
Prototype | void SMBus1_Close(); |
---|---|
Returns | Nothing. |
Description | Closes SMBus connection. |
Requires | SMBus must be configured before using this function. See SMBus1_Init. |
Example |
Library Example
This code demonstrates use of SMBus Library procedures and functions. 8051 MCU is connected (SCL, SDA pins ) to 24c02 EEPROM. Program sends data to EEPROM (data is written at address 2). Then, we read data via SMBus from EEPROM and send its value to PORTA, to check if the cycle was successful. Check the figure below.
HW Connection
Interfacing 24c02 to 8051 via SMBus
What do you think about this topic ? Send us feedback!
Released:
smbus2 is a drop-in replacement for smbus-cffi/smbus-python in pure Python
Project description
A drop-in replacement for smbus-cffi/smbus-python in pure Python
smbus2 is (yet another) pure Python implementation of of the python-smbus package.
It was designed from the ground up with two goals in mind:
- It should be a drop-in replacement of smbus. The syntax shall be the same.
- Use the inherent i2c structs and unions to a greater extent than other pure Python implementations like pysmbus does. By doing so, it will be more feature complete and easier to extend.
Currently supported features are:
- Get i2c capabilities (I2C_FUNCS)
- SMBus Packet Error Checking (PEC) support
- read_byte
- write_byte
- read_byte_data
- write_byte_data
- read_word_data
- write_word_data
- read_i2c_block_data
- write_i2c_block_data
- write_quick
- process_call
- read_block_data
- write_block_data
- block_process_call
- i2c_rdwr - combined write/read transactions with repeated start
It is developed on Python 2.7 but works without any modifications in Python 3.X too.
More information about updates and general changes are recorded in the change log.
smbus2 installs next to smbus as the package, so it's not really a 100% replacement. You must change the module name.
Example 1a: Read a byte
Example 1b: Read a byte using 'with'
This is the very same example but safer to use since the smbus will be closed automatically when exiting the with block.
Example 1c: Read a byte with PEC enabled
Same example with Packet Error Checking enabled.
Example 2: Read a block of data
You can read up to 32 bytes at once.
Example 3: Write a byte
Example 4: Write a block of data
It is possible to write 32 bytes at the time, but I have found that error-prone. Write less and add a delay in between if you run into trouble.
Starting with v0.2, the smbus2 library also has support for combined read and write transactions. i2c_rdwr is not really a SMBus feature but comes in handy when the master needs to:
- read or write bulks of data larger than SMBus' 32 bytes limit.
- write some data and then read from the slave with a repeated start and no stop bit between.
Each operation is represented by a i2c_msg message object.
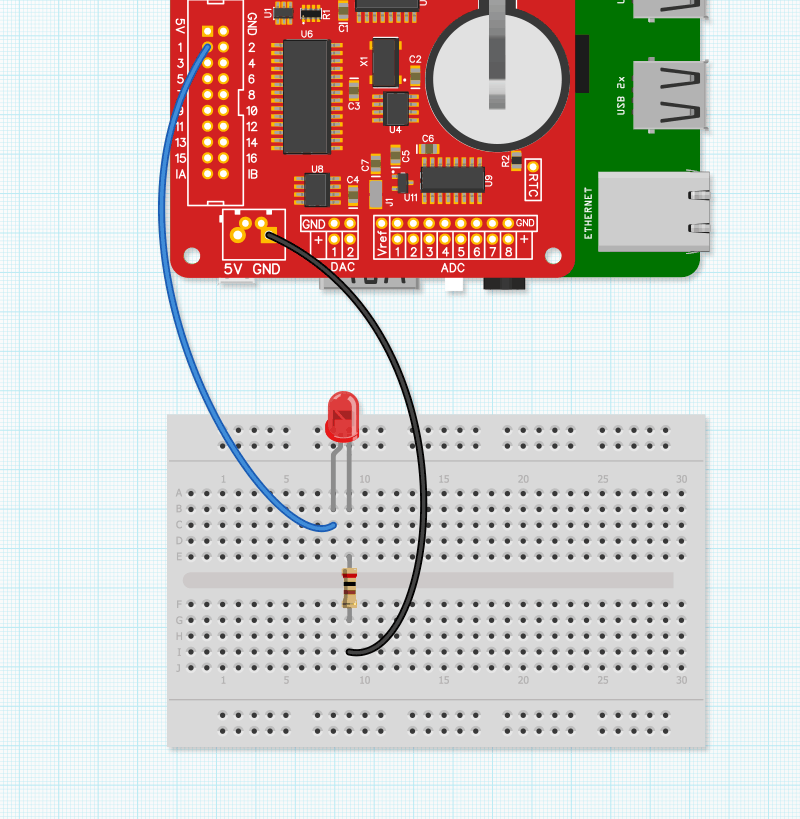
Example 5: Single i2c_rdwr
Example 6: Dual i2c_rdwr
To perform dual operations just add more i2c_msg instances to the bus call:
Example 7: Access i2c_msg data
All data is contained in the i2c_msg instances. Here are some data access alternatives.
From PyPi with pip
:
From conda-forge using conda
:
Installation from source code is straight forward:
Release historyRelease notifications | RSS feed
0.4.1
0.4.0
0.3.0
0.2.3
0.2.2
0.2.1
0.2.0
0.1.5
0.1.4
0.1.3

0.1.2
0.1.1
0.1.0
Download files
Download the file for your platform. If you're not sure which to choose, learn more about installing packages.
Filename, size | File type | Python version | Upload date | Hashes |
---|---|---|---|---|
Filename, size smbus2-0.4.1-py2.py3-none-any.whl (11.5 kB) | File type Wheel | Python version py2.py3 | Upload date | Hashes |
Filename, size smbus2-0.4.1.tar.gz (16.8 kB) | File type Source | Python version None | Upload date | Hashes |
Hashes for smbus2-0.4.1-py2.py3-none-any.whl
Algorithm | Hash digest |
---|---|
SHA256 | 8b1e70cda011b6fb3caf8377a1084f73a5aa99392b78529f140b0a3f06468f6c |
MD5 | 47c994cf8cb7207a922c748fdedfd91c |
BLAKE2-256 | c8bf62ef029fb7077fc87c3539f7365859bccc6cedb2bb20796b737b788c8d09 |
Smbus Library C
CloseHashes for smbus2-0.4.1.tar.gz
Linux Smbus Library
Algorithm | Hash digest |
---|---|
SHA256 | 6276eb599b76c4e74372f2582d2282f03b4398f0da16bc996608e4f21557ca9b |
MD5 | ea4bba25b43863aecd6ec33b2252fdae |
BLAKE2-256 | d933787448c69603eec96af07d36f7ae3a7e3fce4020632eddb55152dc903233 |